How to Use Network Throttling in DevTools to Debug JavaScript in Slow Networks?
The diversified digital landscape of today has users accessing web applications from different devices and varying network conditions, such as slow and unstable internet connectivity. It can be irksome to a user's experience if performance on such slow networks is poor; affecting their interaction and satisfaction in general. Network throttling in DevTools is one critical tool for a developer to simulate these kinds of conditions and effectively debug JavaScript applications. This guide will walk you through how to make use of network throttling to make sure that you find and fix the performance bottlenecks, decrease loading times, and ensure a smooth experience on all networks.
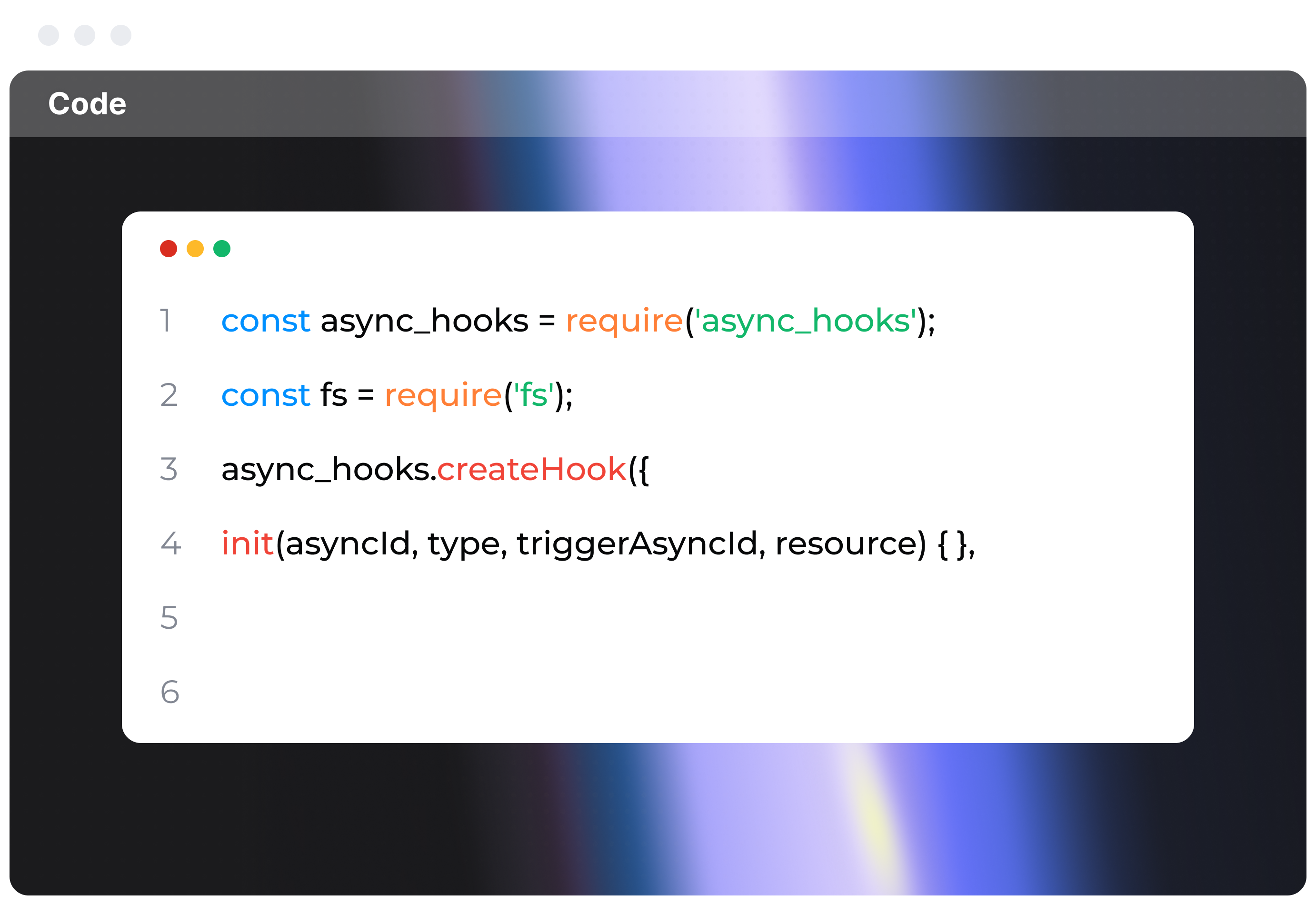
Network Throttling: Why It Matters
Network throttling is a big deal for web developers when it comes to figuring out how well their JavaScript performs under different network conditions. When you simulate slower networks, it helps you see what your users experience when their internet connection isn't great. So, how about we dive into how to use network throttling in DevTools to debug JavaScript on sluggish networks?
Open DevTools:
- In Chrome, just right-click on any spot on the webpage you want to test and choose "Inspect," or you can hit
Ctrl+Shift+I
if you're on Windows or Linux. For Mac users, it's Cmd+Opt+I
.
Go to the Network Tab:
- After DevTools pops up, click on the "Network" tab, which you'll find at the top.
Find the Throttling Menu:
- Look in the Network tab for the throttling dropdown menu at the top of the panel. It usually says "Online" by default.
- Click the dropdown arrow next to "Online" and you'll see presets like "Offline", "GPRS", "Regular 2G", "Good 2G", "Regular 3G", "Good 3G", "Regular 4G", "DSL", and "WiFi."
Choose a Throttling Preset:
- Pick a slower network setting, maybe "Regular 3G." This one makes your network act like a typical 3G connection, with a 300ms delay and max speeds of 780kbps for both download and upload.
Reload the Page:
- Once you've set your throttling, reload the page. You can either press
F5
or hit the refresh button. This makes sure all the network requests are captured under the simulated conditions.
Check Out Network Requests:
- Watch the network requests in the Network tab. You'll see loading times are longer, helping you spot bottlenecks like large files or inefficient network requests.
Debug JavaScript:
- With your page loaded under these conditions, switch to the "Sources" tab to start debugging your JavaScript.
- You can set breakpoints, step through your code, and analyze how different functions and operations perform. Just click on the line number to set a breakpoint.
Monitor Performance:
- Head over to the "Performance" tab to take a performance trace while the page is loading.
- Click the record button (looks like a circle), reload your page, wait for it to fully load, then stop the recording.
- Check out the performance trace to find any Javascript execution bottlenecks and see how long scripts take to run, affecting the overall load time.
Debugging JavaScript Loading: Example
Let's say you have a JavaScript function that's fetching some data from an API and processing it. You think this might be causing issues when the network's slow. Take a look at this example:
function fetchData() {
const url = 'https://api.example.com/data';
// Perform network request
fetch(url)
.then(response => response.json())
.then(data => {
// Process data
processData(data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}
function processData(data) {
// Simulate data processing
console.log('Processing data:', data);
for (let i = 0; i < data.length; i++) {
const item = data[i];
console.log('Item', i, ':', item);
}
}
Set Throttling:
- Open DevTools, go to the Network tab, and choose "Regular 3G."
Set Breakpoints:
- Go to the "Sources" tab and find the JavaScript file with the
fetchData
function.
- Set a breakpoint at the start of the
then
block where processData(data)
is called.
Reload the Page:
- Reload the page to make sure the fetch request happens under the simulated slow network conditions.
- DevTools will pause when it hits the breakpoint.
Step and Analyze:
- Use the step-over (
F10
) and step-into (F11
) buttons to walk through your code and see how long each part takes to execute.
Performance Trace:
- Switch to the "Performance" tab and record a performance trace while the page reloads.
- Once you have the data, analyze the trace to find any unexpected delays or performance bottlenecks.
Making Custom Throttling Profiles
If the built-in profiles don't suit your needs, you can create custom ones.
Open Custom Settings:
- Click the three-dot menu icon in the Network tab.
- Select "Network Conditions."
Create a Custom Profile:
- Scroll to the "Custom" section.
- Enter the download/upload speeds and latency you want to simulate.
Conclusion
Network throttling in DevTools is awesome for debugging JavaScript. It helps you see how your web application performs under various network conditions. By mimicking slower networks, you can find performance bottlenecks, optimize data fetching and processing, and give your users a better experience.
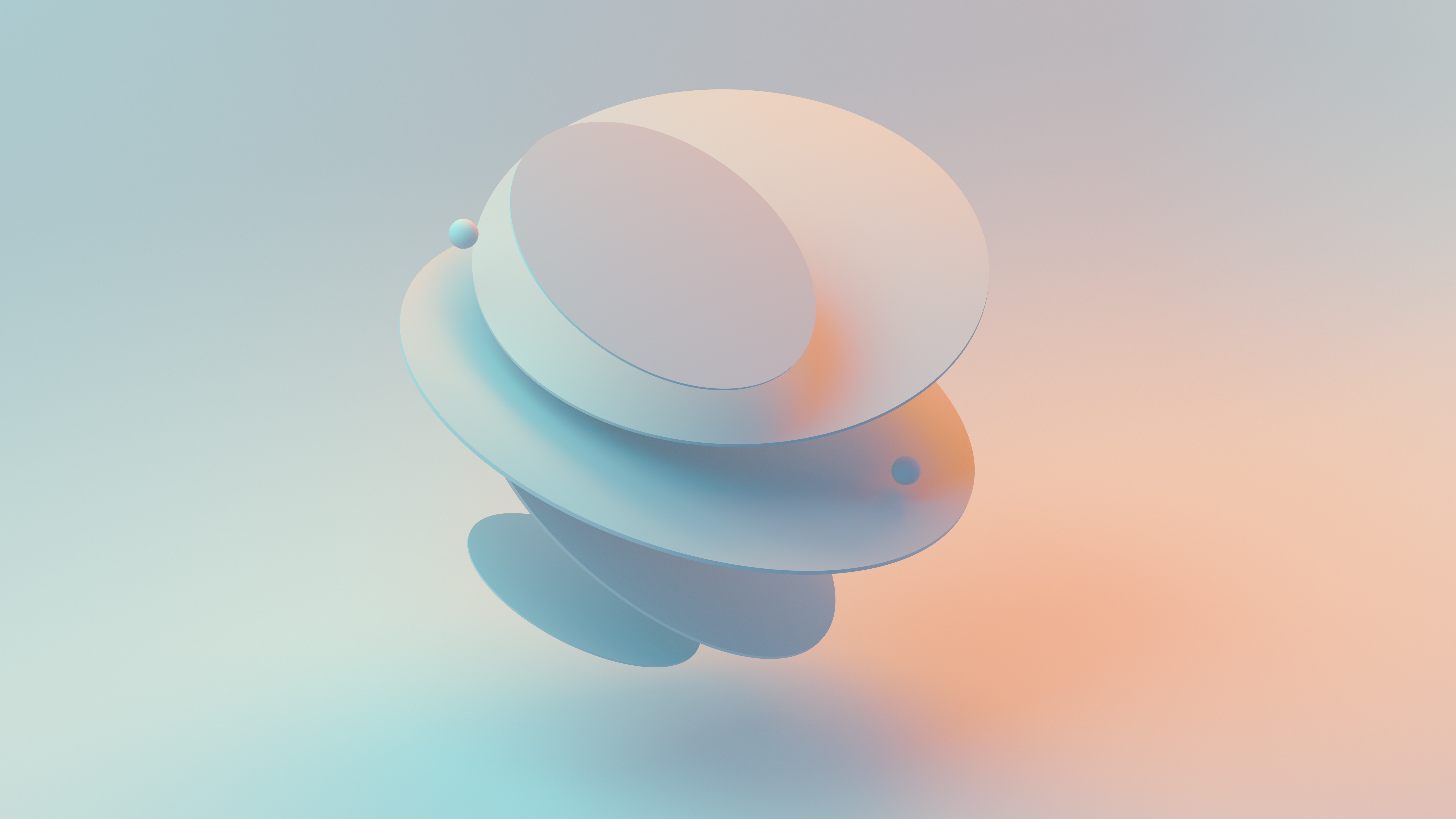
July 21, 2024
How to Use Network Throttling in DevTools to Debug JavaScript in Slow Networks?
Content verified by Anycode AI
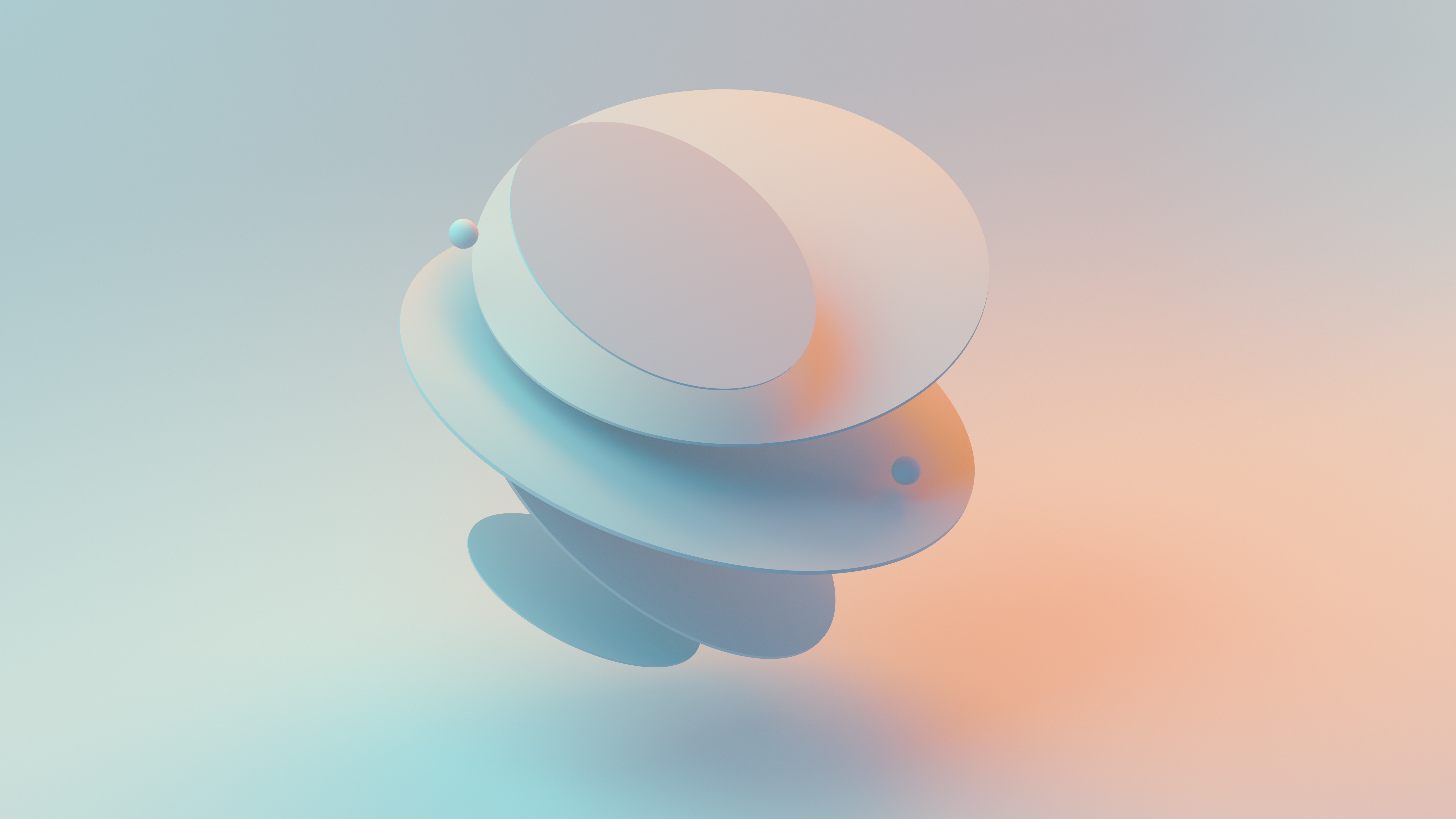
July 21, 2024
How to Use Network Throttling in DevTools to Debug JavaScript in Slow Networks?
Content verified by Anycode AI